如何进行桌面提醒编程?
桌面提醒是一种常见的功能,可以通过弹出窗口、发送通知等方式,在用户使用电脑的过程中提醒用户重要的事件或信息。在编程领域,实现桌面提醒通常涉及到图形用户界面(GUI)的编程和操作系统的API调用。
下面将介绍几种常见的桌面提醒编程方式和相关的技术。
1. Windows 平台
Windows平台可以使用C、C 或者PowerShell等编程语言实现桌面提醒。下面是一个使用C编写桌面提醒的示例:
```csharp
using System;
using System.Windows.Forms;
namespace DesktopAlert
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
MessageBox.Show("这是一个桌面提醒!");
}
}
}
```
通过上述代码,当点击按钮的时候,会弹出一个桌面提醒的窗口。
2. macOS 平台
在macOS平台下,可以使用ObjectiveC或者Swift编程语言实现桌面提醒。下面是一个使用ObjectiveC编写桌面提醒的示例:
```objectivec
import
import
int main(int argc, const char * argv[]) {
@autoreleasepool {
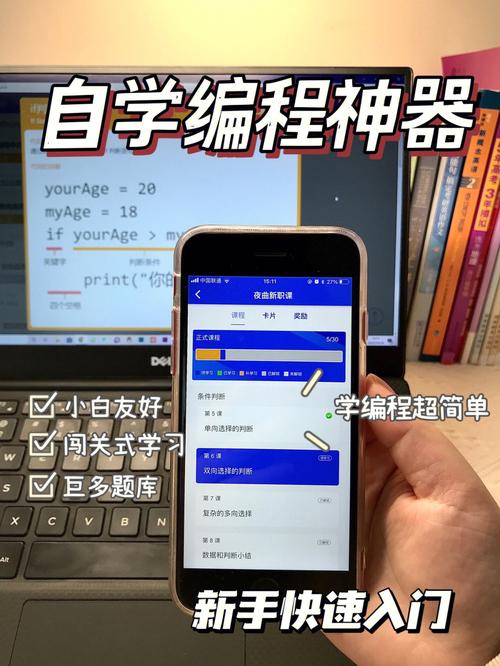
UNUserNotificationCenter* center = [UNUserNotificationCenter currentNotificationCenter];
UNMutableNotificationContent* content = [[UNMutableNotificationContent alloc] init];
content.title = @"桌面提醒";
content.body = @"这是一个桌面提醒!";
content.sound = [UNNotificationSound defaultSound];
UNTimeIntervalNotificationTrigger* trigger = [UNTimeIntervalNotificationTrigger
triggerWithTimeInterval:5 repeats:NO];
UNNotificationRequest* request = [UNNotificationRequest requestWithIdentifier:@"DesktopAlert"
content:content trigger:trigger];
[center addNotificationRequest:request withCompletionHandler:^(NSError * _Nullable error) {
if (error != nil) {
NSLog(@"添加桌面提醒失败:%@", error.localizedDescription);
}
}];
[[NSRunLoop currentRunLoop] run];
}
return 0;
}
```
通过上述代码,会在5秒后发送一个桌面提醒。
3. Linux 平台
Linux平台下,可以使用Python、GTK或者Qt等工具库实现桌面提醒。下面是一个使用Python和PyGObject库编写桌面提醒的示例:
```python
import gi
gi.require_version('Notify', '0.7')
from gi.repository import Notify
Notify.init("Desktop Alert")
notification = Notify.Notification.new("桌面提醒", "这是一个桌面提醒")
notification.show()
```
通过上述代码,会弹出一个桌面提醒。
在实际开发中,桌面提醒还可以结合操作系统的API调用实现更复杂的功能,如显示图标、播放声音、弹出系统通知等。要考虑用户的使用体验,避免过多的提醒或者弹窗对用户造成困扰。
总结一下,桌面提醒编程是一种常见的功能,可以通过使用各种编程语言和相应的工具库,结合操作系统的API调用实现。通过编程,我们可以实现各种形式的桌面提醒,提升用户的使用体验。