迷宫游戏编程创作过程
Title: Programming a Maze Game: A Beginner's Guide
Creating a maze game, like "Migong," can be an exciting project for beginner programmers. It involves elements of game design, graphics, and logic. In this guide, we'll explore the basic steps to program a simple maze game using a popular programming language like Python.
Setting Up the Environment
Before diving into coding, make sure you have the necessary tools set up:
1.
Programming Language:
Choose a language suitable for game development. Python is a great choice due to its simplicity and readability.2.
Integrated Development Environment (IDE):
Install an IDE such as PyCharm or Visual Studio Code, which provides features like syntax highlighting and debugging.3.
Graphics Library:
For creating graphics, consider using libraries like Pygame, which simplifies game development tasks.Designing the Game
Before writing code, it's essential to have a clear understanding of the game's mechanics and structure:
1.
Maze Generation:
Decide how you'll generate the maze. You can use algorithms like recursive backtracking or depthfirst search to create random mazes.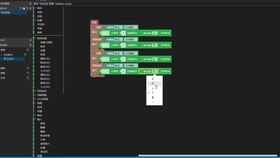
2.
Player Control:
Determine how the player will navigate the maze. This typically involves keyboard input to move the player character (e.g., arrow keys).3.
Goal:
Define the objective of the game. In Migong, it's usually to reach a specific point in the maze.4.
Graphics:
Plan the visual elements of the game, including the maze walls, player character, and goal marker.Writing the Code
Now, let's dive into coding the game. Below is a simplified example using Python and Pygame:
```python
import pygame
import random
Constants
WIDTH, HEIGHT = 800, 600
CELL_SIZE = 40
WHITE = (255, 255, 255)
BLACK = (0, 0, 0)
Initialize Pygame
pygame.init()
screen = pygame.display.set_mode((WIDTH, HEIGHT))
clock = pygame.time.Clock()
Maze Generation
def generate_maze():
Maze generation code goes here (e.g., recursive backtracking)
Player Class
class Player:
def __init__(self, x, y):
self.x = x
self.y = y
def move(self, dx, dy):
Move player if the new position is not a wall
pass
def draw(self):
Draw player character
pass
Game Loop
def main():
player = Player(0, 0)
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
Handle player movement input
Update game state
Draw everything
screen.fill(WHITE)
Draw maze
Draw player
pygame.display.flip()
clock.tick(30)
if __name__ == "__main__":
main()
```
Testing and Refinement
Once the basic functionality is implemented, test the game thoroughly to identify bugs and areas for improvement. Consider adding features like multiple levels, scoring, or enemy characters to enhance gameplay.
Conclusion
Programming a maze game like Migong is an excellent way for beginners to learn game development concepts. By following this guide and experimenting with the code, you can create your own engaging maze game from scratch. Happy coding!