Title: A Beginner's Guide to SSHC Programming
SSH (Secure Shell) is a cryptographic network protocol for secure data communication, remote commandline login, remote command execution, and other secure network services between two networked computers. SSHC (Secure Shell Copy) is an extension of SSH, primarily used for securely copying files between computers. Programming with SSHC involves automating file transfers securely between systems. Here's a beginner's guide to SSHC programming:
Understanding SSHC:
SSH is commonly used for securely accessing remote systems and executing commands on them. SSHC extends this functionality to securely copying files between systems. SSHC utilizes the SSH protocol for secure authentication and data transfer.
Setting Up SSHC:
Before programming with SSHC, ensure SSH is properly configured on both the source and destination systems. This involves generating SSH keys, configuring SSH access, and ensuring proper permissions are set up.
Choosing a Programming Language:
SSH libraries are available for various programming languages, including Python, Java, and Ruby. Choose a language that best suits your project requirements and your familiarity with the language.
Python Example:
```python
import paramiko
SSHC function to copy a file from source to destination
def sshc_copy(source_file, destination_file, hostname, username, password):
Create SSH client
ssh = paramiko.SSHClient()
ssh.set_missing_host_key_policy(paramiko.AutoAddPolicy())
Connect to the SSH server
ssh.connect(hostname, username=username, password=password)
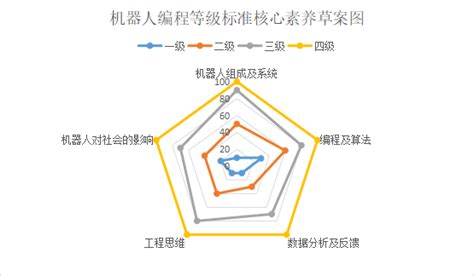
Use SFTP to transfer files
sftp = ssh.open_sftp()
Copy file from source to destination
sftp.put(source_file, destination_file)
Close SFTP connection
sftp.close()
Close SSH connection
ssh.close()
Example usage
sshc_copy('local_file.txt', '/remote/path/destination_file.txt', 'hostname', 'username', 'password')
```
Java Example:
```java
import com.jcraft.jsch.*;
public class SSHCExample {
// SSHC method to copy a file from source to destination
public static void sshcCopy(String sourceFile, String destinationFile, String hostname, String username, String password) throws JSchException, SftpException {
JSch jsch = new JSch();
Session session = jsch.getSession(username, hostname, 22);
session.setPassword(password);
session.setConfig("StrictHostKeyChecking", "no");
session.connect();
ChannelSftp channelSftp = (ChannelSftp) session.openChannel("sftp");
channelSftp.connect();
// Copy file from source to destination
channelSftp.put(sourceFile, destinationFile);
channelSftp.disconnect();
session.disconnect();
}
// Example usage
public static void main(String[] args) throws JSchException, SftpException {
sshcCopy("local_file.txt", "/remote/path/destination_file.txt", "hostname", "username", "password");
}
}
```
Recommendations:
Security
: Always prioritize security when using SSHC. Ensure proper authentication methods are used and sensitive information like passwords are handled securely.
Error Handling
: Implement robust error handling in your code to gracefully handle connection failures, authentication errors, or file transfer issues.
Testing
: Thoroughly test your SSHC code in various scenarios to ensure reliability and proper functionality.
Documentation
: Document your SSHC code comprehensively to facilitate maintenance and future development.By following this guide and using the provided examples, you can start programming with SSHC to securely transfer files between systems in your projects. Remember to adapt the code to fit your specific requirements and incorporate best practices for security and reliability.